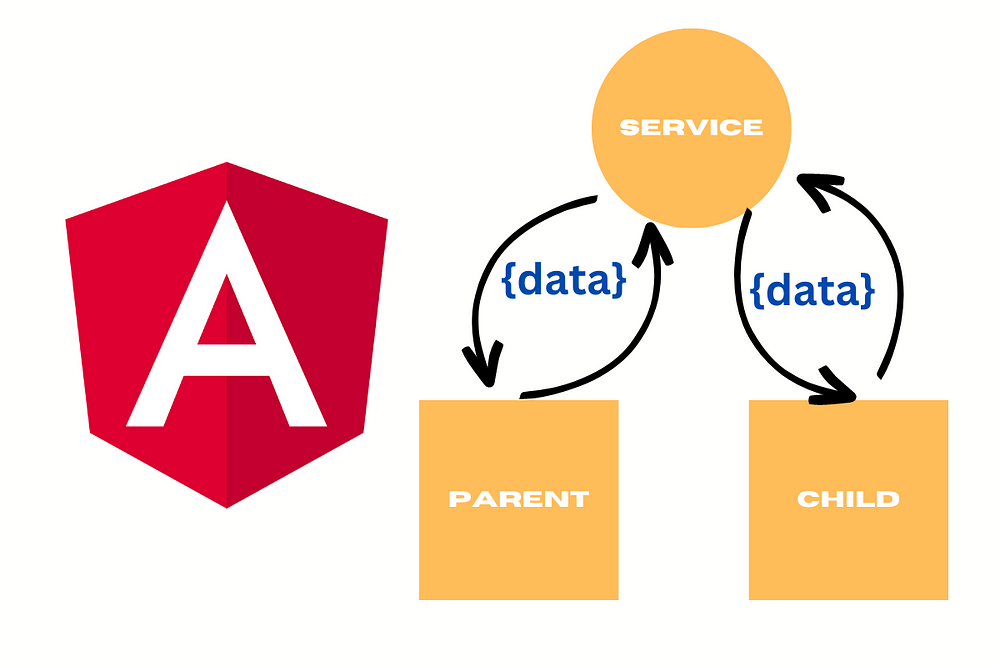
Here’s an example of how to pass a variable from a child component to a parent component using a shared service in Angular:
1. Create a shared service
import { Injectable } from '@angular/core';
import { Subject } from 'rxjs';
@Injectable({
providedIn: 'root'
})
export class DataService {
private dataSource = new Subject<any>();
data$ = this.dataSource.asObservable();
sendData(data: any) {
this.dataSource.next(data);
}
}
In the above example, we are creating a shared service called DataService
that will hold the data. The dataSource
is an RxJS Subject
and data$
is an observable that we can subscribe to. The sendData
method is used to send the data to the subscribers.
2. Inject the service into the child component
import { Component } from '@angular/core';
import { DataService } from './data.service';
@Component({
selector: 'app-child',
template: `
<button (click)="sendData()">Send Data</button>
`
})
export class ChildComponent {
constructor(private dataService: DataService) {}
sendData() {
this.dataService.sendData('Hello from the child component!');
}
}
In the child component, we are injecting the DataService
and using the sendData
method to send the data.
3. Inject the service into the parent component and subscribe to the data
import { Component } from '@angular/core';
import { DataService } from './data.service';
@Component({
selector: 'app-parent',
template: `
<app-child></app-child>
`
})
export class ParentComponent {
constructor(private dataService: DataService) {
this.dataService.data$.subscribe(data => {
console.log(data);
});
}
}
In the parent component, we are also injecting the DataService
and subscribing to the data$
observable. In the subscribe block we can handle the data received from the child component.
In this example, when the button in the child component is clicked, it sends the data to the parent component using the shared service. The parent component is able to receive the data by subscribing to the service’s observable.
This way, we can share data between multiple components in a consistent manner and make it more reusable.